Handling Errors
No error handling
What happens when you render a page that throws an error? Let's find out!
What you see will depend on whether you are running in dev or production mode. Here's what dev looks like:
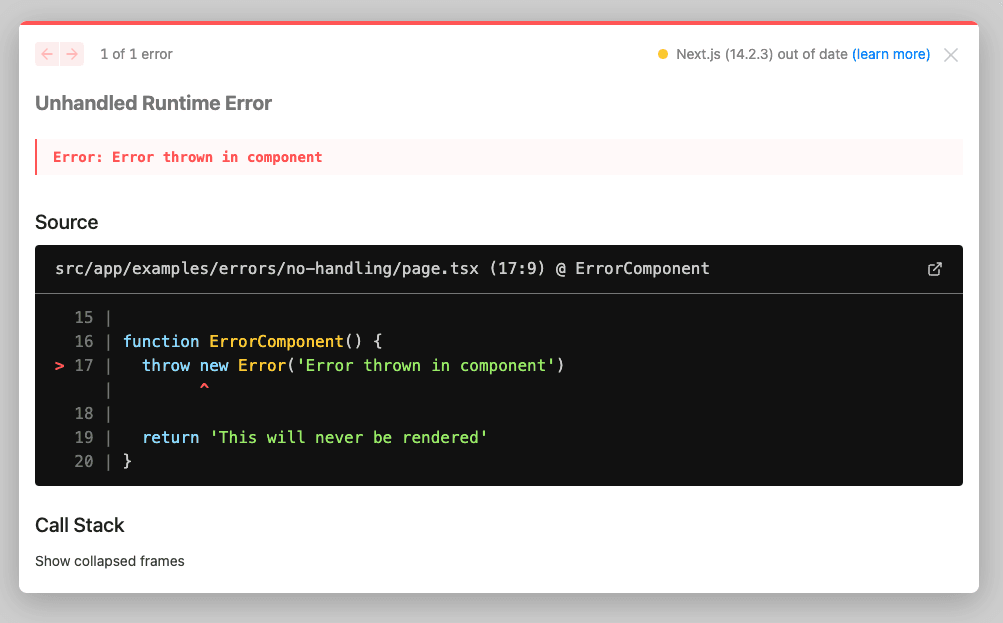
And here's what production looks like:
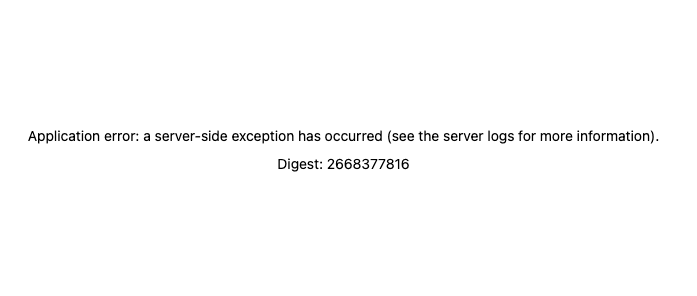
That's not the greatest user experience in the world, and even though only one component in our app is throwing an error, the entire app is affected. Let's see how we can improve this with either an explicit error boundary or an error.tsx.
Live Demo
This example can't be easily inlined as it demonstrates how a full-page feels to the end user. Here it is inside an iframe, and there's a looping video below too.